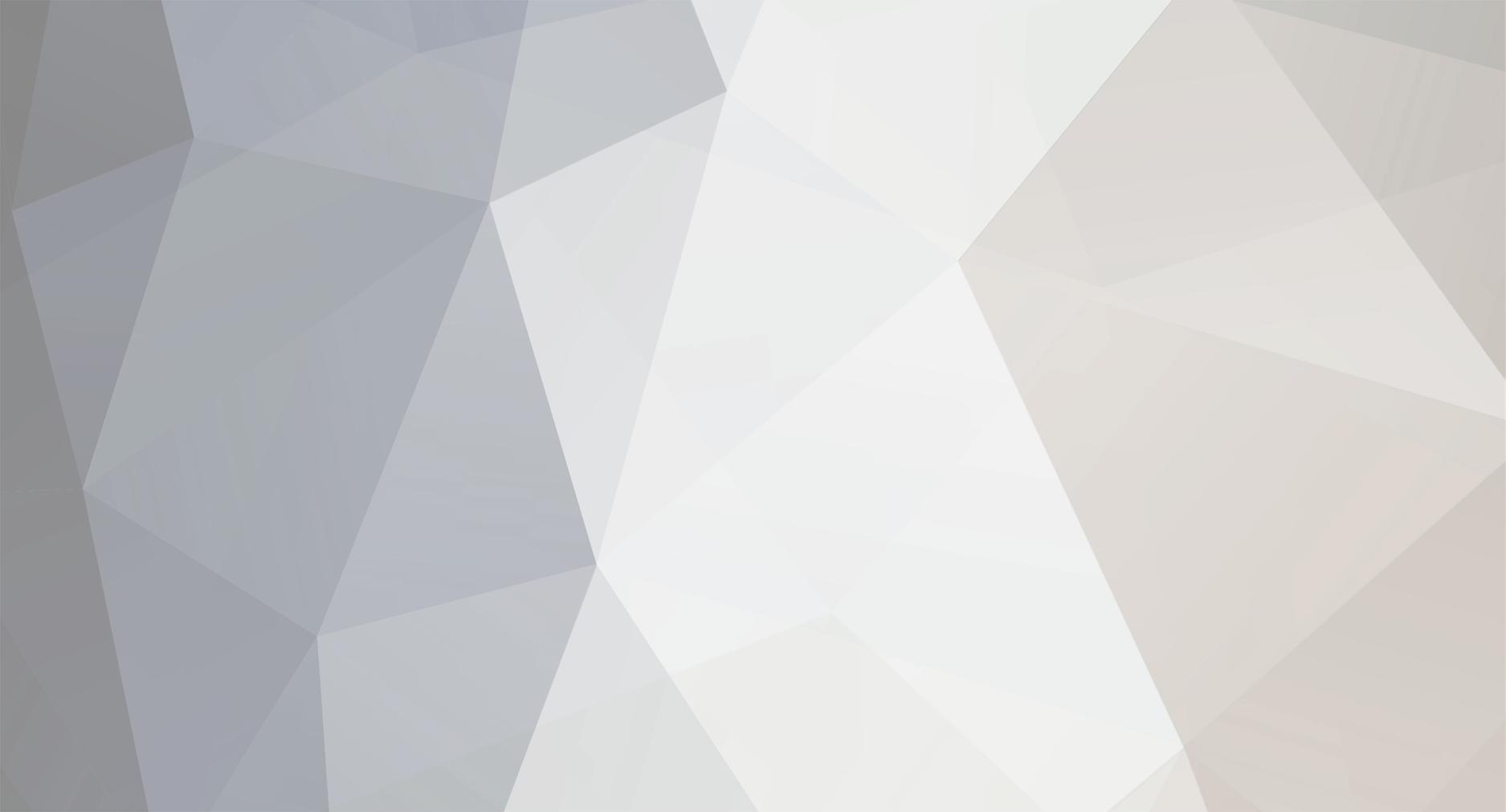
olek07
-
Gesamte Inhalte
123 -
Benutzer seit
-
Letzter Besuch
Inhaltstyp
Profile
Forum
Downloads
Kalender
Blogs
Shop
Beiträge von olek07
-
-
Hallo zusammen,
wieviel kann ich als Webentwickler mit mehrjähriger Berufserfahrung verlangen? 55000 € pro Jahr?
Vielen Dank.
-
Hallo zusammen,
ich versuche ein Programm zu schreiben, dass eine gerade heruntergeladene Datei in den vorgegebenen Ordner verschiebt und durch eine andere vorhandene Datei ersetzt. Das Programm muss auf Windows-Maschine laufen. Ist so was mit Java möglich?
Ich habe mit dem Watcher probiert, aber es funktioniert nicht wirklich.
Vielen Dank für eure Hilfe
import java.nio.file.*; import static java.nio.file.StandardWatchEventKinds.*; import static java.nio.file.LinkOption.*; import java.nio.file.attribute.*; import java.io.*; import java.util.*; import java.util.regex.*; /** * Example to watch a directory (or tree) for changes to files. */ public class WatchDir { private final WatchService watcher; private final Map<WatchKey,Path> keys; private final boolean recursive; private boolean trace = false; @SuppressWarnings("unchecked") static <T> WatchEvent<T> cast(WatchEvent<?> event) { return (WatchEvent<T>)event; } /** * Register the given directory with the WatchService */ private void register(Path dir) throws IOException { WatchKey key = dir.register(watcher, ENTRY_CREATE, ENTRY_DELETE, ENTRY_MODIFY); if (trace) { Path prev = keys.get(key); if (prev == null) { System.out.format("register: %s\n", dir); } else { if (!dir.equals(prev)) { System.out.format("update: %s -> %s\n", prev, dir); } } } keys.put(key, dir); } /** * Register the given directory, and all its sub-directories, with the * WatchService. */ private void registerAll(final Path start) throws IOException { // register directory and sub-directories Files.walkFileTree(start, new SimpleFileVisitor<Path>() { @Override public FileVisitResult preVisitDirectory(Path dir, BasicFileAttributes attrs) throws IOException { register(dir); return FileVisitResult.CONTINUE; } }); } /** * Creates a WatchService and registers the given directory */ WatchDir(Path dir, boolean recursive) throws IOException { this.watcher = FileSystems.getDefault().newWatchService(); this.keys = new HashMap<WatchKey,Path>(); this.recursive = recursive; if (recursive) { System.out.format("Scanning %s ...\n", dir); registerAll(dir); System.out.println("Done."); } else { register(dir); } // enable trace after initial registration this.trace = true; } /** * Process all events for keys queued to the watcher */ void processEvents() { boolean nowCopied = false; for (; { // wait for key to be signalled WatchKey key; try { key = watcher.take(); } catch (InterruptedException x) { return; } Path dir = keys.get(key); if (dir == null) { System.err.println("WatchKey not recognized!!"); continue; } for (WatchEvent<?> event: key.pollEvents()) { WatchEvent.Kind kind = event.kind(); // TBD - provide example of how OVERFLOW event is handled if (kind == OVERFLOW) { continue; } // Context for directory entry event is the file name of entry WatchEvent<Path> ev = cast(event); Path name = ev.context(); Path child = dir.resolve(name); // print out event // System.out.format("%s: %s\n", event.kind().name(), child); //if (kind == ENTRY_CREATE) if (kind != ENTRY_DELETE) { Pattern p = Pattern.compile("2013-10-.*-evn\\.pdf$", Pattern.CASE_INSENSITIVE); Matcher m = p.matcher(child.toString()); if (m.find()) { System.out.println("nowCopied: " + nowCopied + "\n"); if (!nowCopied) { System.out.println("matches"); File f = new File(child.toString()); System.out.print(f.delete()); Path copySourcePath = Paths.get("d:\\aaa.txt"); nowCopied = true; try { Thread.sleep(8000); System.out.print("Jetzt wird kopiert\n"); Files.copy(copySourcePath, child); System.exit(0); } catch (IOException e) { } catch (InterruptedException e) { } finally { } nowCopied = false; } } } // if directory is created, and watching recursively, then // register it and its sub-directories if (recursive && (kind == ENTRY_CREATE)) { try { if (Files.isDirectory(child, NOFOLLOW_LINKS)) { registerAll(child); } } catch (IOException x) { // ignore to keep sample readbale } } } // reset key and remove from set if directory no longer accessible boolean valid = key.reset(); if (!valid) { keys.remove(key); // all directories are inaccessible if (keys.isEmpty()) { break; } } } } static void usage() { System.err.println("usage: java WatchDir [-r] dir"); System.exit(-1); } public static void main(String[] args) throws IOException { // parse arguments if (args.length == 0 || args.length > 2) usage(); boolean recursive = false; int dirArg = 0; if (args[0].equals("-r")) { if (args.length < 2) usage(); recursive = true; dirArg++; } // register directory and process its events Path dir = Paths.get(args[dirArg]); new WatchDir(dir, recursive).processEvents(); } } [/code]
-
es werden einige Klassen importiert.
import org.apache.xmlrpc.client.XmlRpcClient; import org.apache.xmlrpc.client.XmlRpcClientConfigImpl;
Die jars werden hier benutzt:
ws-commons-util.jar
xmlrpc-client.jar
xmlrpc-common.jar
Leider ist die Dokumentation dazu nicht wirklich hilfreich. http://ws.apache.org/xmlrpc/client.html
client wird so definiert: XmlRpcClient client = new XmlRpcClient(); und liefert ein Objekt. result kann man im Prinzip auch so definieren:
HashMap result = new HashMap();
result = (HashMap) client.execute("get_thread", params);
System.out.println(result.get("forum_id"));
Gibt es keine allgemein Lösung, wie man Objekte aus dem Objekt auslesen kann?
-
und wie kann ich aus topic_title einen String gewinnen? System.out.println(result) liefert nur "topic_title=[b@1277a30}"
-
es geht hier um die Response einer XMLRPC-Abfrage. Also es gibt leider keine Getter. (ist doch nicht möglich bei XMLRPC, oder?)
Ich kann toString überschreiben, aber zu welchem Zweck? Und in der überschriebenen Methode brauche ich auch die Möglichkeit auf die Members zuzugreifen. Ich habe die Lösung mit HashMap gefunden, allerdings bin ich damit nicht sehr zufrieden. Der Compiler sagt:
Note: XmlRpcTest.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
was ganz logisch ist. Und einige Members des Response-Objectes sind selbst Objekte. Also da brächte ich wieder eine Hashmap. Ich habe keine andere Idee.
-
ich habe folgendes mit HashMap gemacht:
HashMap<String, Object> result = new HashMap<String, Object>(); result = (HashMap) client.execute("get_thread", params); System.out.println(result.get("forum_id"));
es funktioniert zwar, aber gibt es eine bessere Lösung?
-
Hallo zusammen,
über eine XMLRPC-Schnittstelle bekomme ich ein Object
Object result = client.execute("get_thread", params);
System.out.println(result) gibt folgendes aus:
{forum_id=16, prefix=[B@34f445, forum_name=[B@90ed81, posts=[Ljava.lang.Object;@d8c3ee, can_upload=false, is_s ubscribed=false, topic_id=3433, total_post_num=131, topic_title=[B@1277a30}
Wie kann ich auf einzelne Werte von forum_id , total_post_num etc zugreifen?
Vielen Dank.
-
ist schon ok. Aber zeigt das obere Beispiel von pr0gg3r eine typische Anwendung von Servlets? Oder wo ist hier der Vorteil.
-
ein Redirect kann auch ohne Servlet gemacht werden.
In JSP kannst du folgendes schreiben:
String site = new String("http://www.photofuntoos.com"); response.setStatus(response.SC_MOVED_TEMPORARILY); response.setHeader("Location", site);
Also response ist auch hier zugänglich. Und das ist im Prinzip ähnlich wie in php. Du rufst bestimmte Methoden auf, mir derer Hilfe die Weiterleitung gemacht wird.
-
Hallo zusammen,
ich komme aus der PHP-Welt und möchte jetzt für mich einige Sachen zu JSP/Servlets klären. Wafür braucht man eingentlich die Servlets? JSP funktioniert ähnlich wie php. Mit JSP kann man alles so gut realisieren wie mit Servlets (oder stimmt es nicht?)
Ein Beispiel-Servlet sieht so aus:
public class HelloWorldExample extends HttpServlet { private static final long serialVersionUID = 1L; @Override public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException { ResourceBundle rb = ResourceBundle.getBundle("LocalStrings",request.getLocale()); response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.println("<html>"); out.println("<head>"); String title = rb.getString("helloworld.title"); out.println("<title>" + title + "</title>"); out.println("</head>"); out.println("<body bgcolor=\"white\">"); // note that all links are created to be relative. this // ensures that we can move the web application that this // servlet belongs to to a different place in the url // tree and not have any harmful side effects. // XXX // making these absolute till we work out the // addition of a PathInfo issue out.println("<a href=\"../helloworld.html\">"); out.println("<img src=\"../images/code.gif\" height=24 " + "width=24 align=right border=0 alt=\"view code\"></a>"); out.println("<a href=\"../index.html\">"); out.println("<img src=\"../images/return.gif\" height=24 " + "width=24 align=right border=0 alt=\"return\"></a>"); out.println("<h1>" + title + "</h1>"); out.println("</body>"); out.println("</html>"); } }
aber mit JSP kann man es dasselbe mit weniger Schreiberei machen.
Danke für die Erklärung.
-
Agenburbereich ist natürlich nicht das beste für mich das stimmt. Web-Branche? naja. Sicher könnte ich auch im anderen Bereich arbeiten. Web macht mir Spass.
-
lass uns bitte nicht über Drupal diskutieren, das nicht einmal OOP mächtig ist.
Ich glaube, JAVA entspicht heutigen Anforderungen an Sicherheit, Geschwindigkeit und Qualität. Habe ich recht?
-
danke für den Hinweis. Projekt-Management will ich nicht. Meiner Meinung nach, wer nicht gut programmieren kann, wird zum Projektmanager. Software-Architektur. ja, es klingt gut. allerdings muss man noch ein bisschen Erfahrung in Java-Programmierung sammeln.
-
eben nicht. denn ich halte php nicht für eine echte Programmiersprache. JSP/Java macht mir bestimmt mehr spass. Es ist halt die Frage, ob es immer noch nachgrfragt wird. Bei der Agentur, wo ich grad arbeite, kannst du es vergessen.
-
mit Klickprogrammierung meine ich vor allem Drupal :-)
-
Hallo zusammen,
ich komme aus der Web-Branche, programmiere seit vielen Jahren in PHP. Letzte Zeit finde ich php ein bisschen langweilig. Lohnt es sich auf JSP umzusteigen? Java finde ich viel interessanter und professioneller, als php. Die Agentur, wo ich arbeite, bieten Kunden nur Drupal/typo3 an. Da ich Diplominformatiker bin, möchte ich richtig Programmieren. Für die "Cklickprogrammierung" bin ich überqualifiziert.
-
Hallo zusammen,
bei mir ist der Fesplatten-Controller kaputtgegangen. Ich möchte die Festplatten-Platine austauschen. Ich habe auf ebay ein paar Platinen gefunden, aber sie sind nicht 100%-ig wie meine. So eine Festplatte habe ich
Seagate Momentus 5400.3 160 GB,Intern,5400 RPM,6,35 cm (2,5 Zoll)
Produktidentifikator
Marke Seagate
Modell Momentus 5400.3
MPN ST9160821AS
EAN 683728169992
Haupteigenschaften
Gehäuse Intern
Kapazität 160 GB
Puffergröße 16 MB
Spindelgeschwindigkeit 5400 RPM
Schnittstelle SATA I
Bezeichnung Desktop-Computer
und das sind die Platiten.
Seagate Momentus 5400.3 PCB HDD Board Sata 2.5" 100GB ST9100828AS 9S113E-065
SCHEDA ELETTRONICA HARD DISK SATA 2.5" DA 100GB
DATI DEL HDD CHE LA MONTAVA
MARCA: SEAGATE
MODELLO: MOMENTUS 5400.3 / ST9100828AS
PN: 9S113E-065
FW: 3.ALB
DATE: 07256
SITE: WU
FATTORE DI FORMA: 2.5"
INTERFACCIA: SATA
DATI DELLA SCHEDA
PN: 100398689 REV B / 100398688 K 3725THLG
und
SEAGATE MOMENTUS 5400.3 ST9120822AS 120GB SATA PCB BOARD ONLY
SEAGATE MOMENTUS 5400.3 ST9120822AS 120GB SATA HARD DRIVE PCB BOARD ONLY
FIRMWARE: 3.CAE
PART NUMBER: 9S1133-042
DATE CODE: 08027
SITE CODE: WU
NUMBER ON THE BACK FOR DATA RECOVERY PURPOSE: 100398689 REV C
PCB BOARD WAS TESTED AND IS IN WORKING CONDITION
Weiß jemand, ob die passen?
Vielen Dank
-
vielen Dank. E hört sich interessant an.
-
ich bin mit diesem Gehalt nicht zufrieden. Das ist mir zu wenig.
Wie kann man die Branche wechseln? Soll ich einen Kurs machen oder gibt es andere Möglichkeiten?
-
das bedeutet die Branche wechseln?
-
Alter: 36
Wohnort: eine große Stadt in Baden-Württemberg
letzter Ausbildungsabschluss Diplominformatiker 1998
Berufserfahrung: 15 Jahre
Vorbildung: Gymnasium
Arbeitsort
Grösse der Firma: 30 MA
Tarif
Branche der Firma: Internetagentur
Arbeitsstunden pro Woche laut Vertrag: 40
Arbeitsstunden pro Woche real: 40
Gesamtjahresbrutto: 28500
Anzahl der Monatsgehälter: 12
Anzahl der Urlaubstage: 24
Verantwortung: keine
Tätigkeiten Technischer Projektleiter, Entwickler
-
ich möchte immer noch in der Web-Branche bleiben. Also Assembler, C++ etc sind weniger gebrauchbar hier.
Ich bin Programmierer mit über 15-jähriger Erfahrung.
-
Vielen Dank. Das werde ich tun!
Ich wohne und arbeite in Baden-Württemberg, Diplominformatiker, Oracle Certified MySQL Developer
-
Hallo zusammen,
ich arbeite seit 2006 als Webentwickler bei einer Firma. Da ich jetzt vor der Entscheidung stehe eine andere Firma zu suchen, möchte ich fragen, was momentan von den Bewerbern erwartet wird. Was soll ich lernen?
Ich bin Diplominformaiker.
Momentan arbeite ich mit PHP, Perl, MySQL (Oracle Certified MySQL Entwickler), HTML, JavaScript (JQuery), Linux, Typo3 (nicht ganz perfekt), Java (kann aber ohne Erfahrung).
Vielen Dank im Voraus.
TYPO3-/PHP5-Entwickler-Position (Lead Developer) im Raum Frankfurt. Wieviel pro Jahr?
in IT-Arbeitswelt
Geschrieben
wofür braucht man das? Ich wollte nur wissen, ob 55 000 Pro Jahr für diese Position normal sind. Ich möchte mich bewerben und muss meine Gehaltsvorstellungen angeben?
Alter: 37
Mehrjährige Berufserfahrung
Diplominformatiker, Oracle Certified Professional MySQL 5 Developer